Securing Data with AWS Key Management Service (KMS)
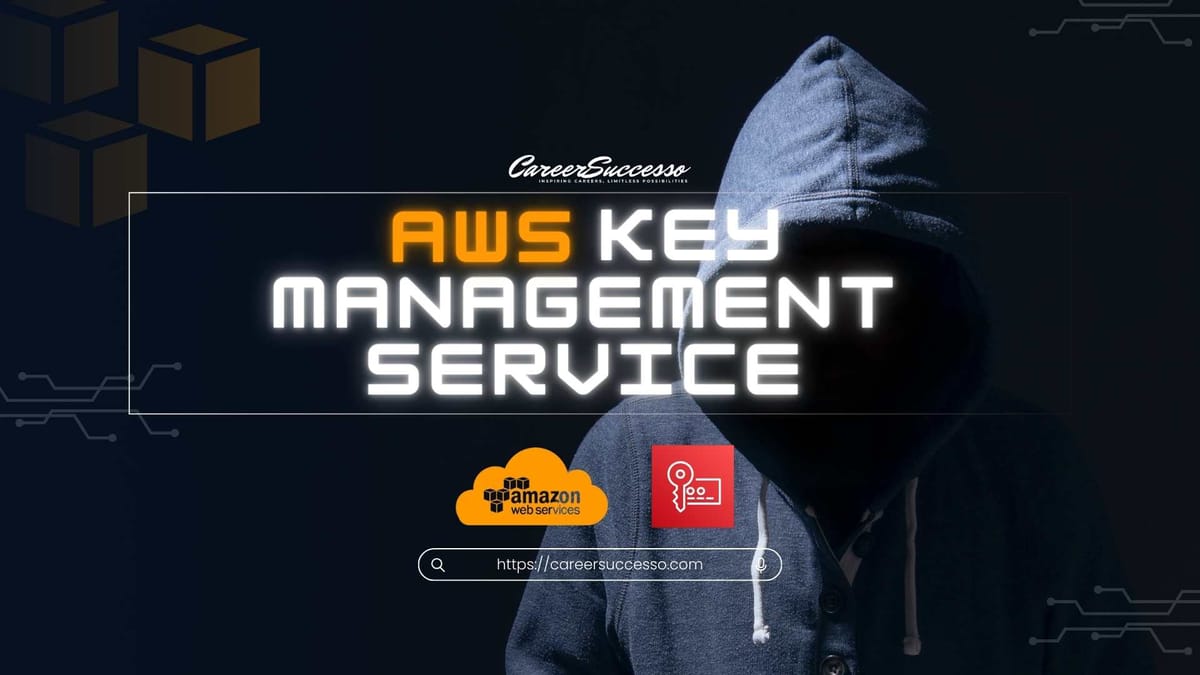
In today's world, ensuring data security is critical for any application. AWS Key Management Service (KMS) provides a simple way to encrypt and decrypt sensitive data. In this post, we'll cover how to use AWS KMS for encrypting and decrypting data, including steps to create a KMS key, and a practical code example that you can find in my GitHub repository.
What is AWS KMS?
AWS KMS is a managed service that makes it easy to create and control encryption keys used to secure your data. KMS integrates with other AWS services to protect data at rest and in transit using hardware security modules (HSMs) to ensure the confidentiality and integrity of your keys.
Key Features:
- Secure Key Storage: Store keys in HSMs for enhanced security.
- Fine-Grained Access Control: Define who can use and manage keys using AWS Identity and Access Management (IAM) policies.
- Automatic Key Rotation: KMS can automatically rotate your keys to enhance security.
- Easy Integration: KMS integrates with multiple AWS services like S3, DynamoDB, and Lambda for data encryption.
KMS Key Types
AWS KMS supports different types of keys to suit various use cases:
1. Symmetric Keys (AES-256)
- Single encryption key: Used for both encryption and decryption.
- Commonly used by AWS services integrated with KMS (e.g., S3, DynamoDB).
- Access control: You never get direct access to the unencrypted key; you must call the KMS API to use it.
2. Asymmetric Keys (RSA & ECC)
- Key pairs: Public (for encryption) and private (for decryption).
- Operations: These keys can also be used for signing and verifying operations.
- Public key: Downloadable for encryption outside AWS, but you can't access the private key unencrypted.
- Use case: Perfect for scenarios where users outside AWS need to encrypt data but cannot call the KMS API.
Types of KMS Keys
AWS KMS offers several types of keys, each with unique management features:
- AWS Owned Keys: Default keys used for services like SSE-S3, SSE-SQS, and SSE-DynamoDB. They’re free and fully managed by AWS.
- AWS Managed Keys: Keys managed by AWS for specific services, such as
aws/rds
oraws/ebs
. These are free but limited to specific use cases. - Customer-Managed Keys: Keys that you create and manage in AWS KMS. These offer more control and flexibility, and cost $1/month.
- Customer-Imported Keys: These keys are brought in from external sources and cost $1/month. However, you need to pay for API calls to KMS ($0.03 per 10,000 calls).
Key Rotation
- AWS Managed Keys: Automatically rotated every year.
- Customer-Managed Keys: Can be configured for automatic rotation (every year) or rotated manually.
- Imported Keys: Must be rotated manually using aliases.
KMS Multi-Region Keys
AWS KMS supports multi-region keys, allowing for global data encryption and decryption without the need for cross-region API calls.
- Identical KMS keys in different AWS Regions can be used interchangeably.
- Same key ID, key material, and automatic rotation across regions.
- Encrypt in one region and decrypt in another, no need to re-encrypt the data.
- Multi-region keys are not global (instead, there are primary and replica keys).
- Use Cases: Perfect for client-side encryption across regions or global services like DynamoDB Global Tables or Aurora Global Databases.
Practical Example: Encrypting and Decrypting Data Using AWS KMS
Steps to Create a KMS Key
Before we dive into the code, let’s create a KMS key that you’ll use for encryption and decryption.
- Open the AWS KMS Console
Log in to your AWS account and go to the AWS KMS Console. - Create a New Key
- Click on Customer managed keys on the left-hand panel.
- Choose Create key.
- Select Symmetric under the Key type section and click Next.
- Under Key usage, choose Encrypt and decrypt.
- Configure the Key
- Give your key an alias, for example,
generatorKey
. - Optionally, add a description to explain the purpose of this key.
- Set Key administrative permissions by selecting IAM users or roles that can manage this key.
- Define Key usage permissions by adding IAM users or roles that can use this key for encryption and decryption.
- Give your key an alias, for example,
- Complete Key Creation
After reviewing the configurations, click Finish. You’ll now have a key available with its Key ID and ARN, which you’ll use in your application.
I’ve prepared a Node.js example that demonstrates how to encrypt and decrypt data using KMS. You can find the complete code in my GitHub repository.
To run the code:
- Install the necessary dependencies:
npm install
- Replace the Key ARN in the code with the ARN of the KMS key you created.
- Run the script using Node.js:
node index.mjs
This will securely encrypt and decrypt your data using the KMS key you created.
Managing Access and Permissions
AWS KMS allows you to define fine-grained access controls using IAM policies. You can specify who can encrypt, decrypt, and manage keys, ensuring that your sensitive data remains secure.
Conclusion
AWS KMS simplifies encryption and decryption, making it an ideal solution for securing sensitive data across AWS services. Whether you're building an application or securing communication, KMS provides a robust, scalable solution.
Check out the complete code example on my GitHub repository for hands-on practice. Let me know how you’re using KMS in your projects!