How to Manage Large Data Writes in DynamoDB
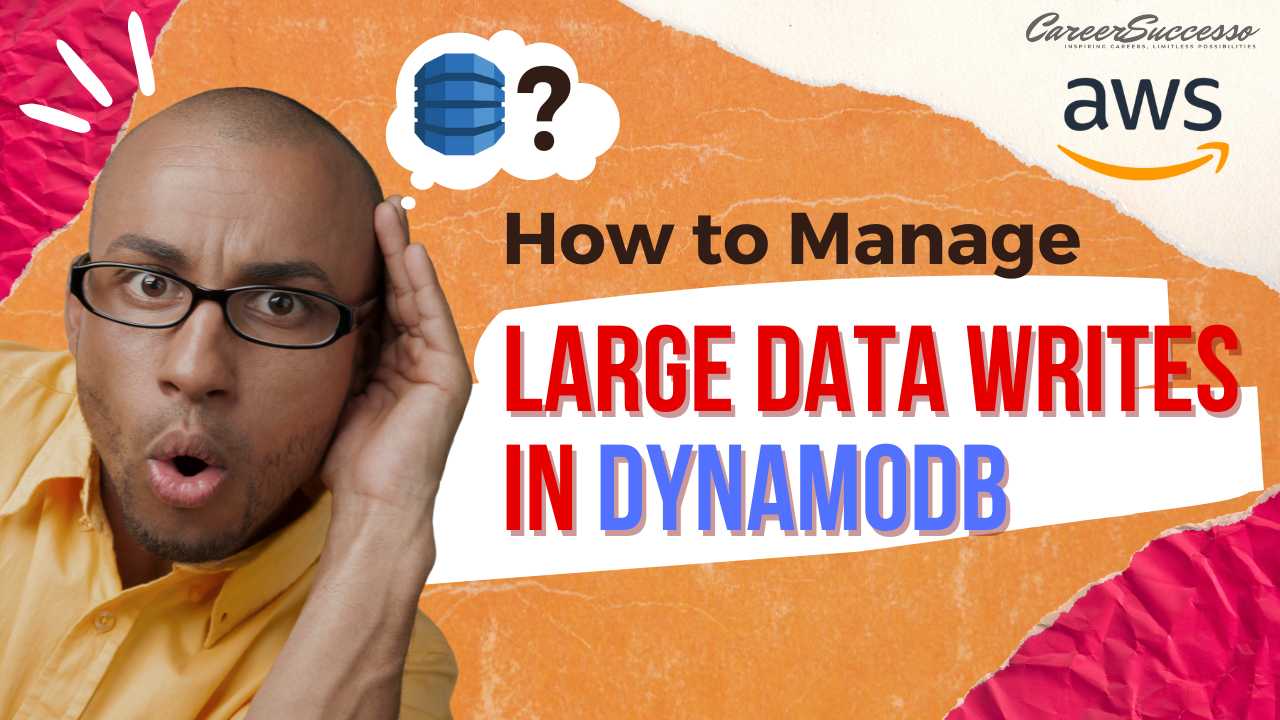
When dealing with a large number of data records in DynamoDB, it’s crucial to handle the operations efficiently to avoid errors and throttling. One effective way is to use the DynamoDB batch write operation. This post will guide you through the process, including handling retries for common errors using an exponential backoff algorithm.
Understanding DynamoDB Batch Write
DynamoDB’s batch write operation allows you to insert multiple items in a single request, up to a maximum of 25 items. However, DynamoDB may occasionally throw errors due to various constraints, necessitating retries. Implementing a backoff algorithm to handle these retries can prevent overwhelming the database and manage write capacity effectively.
Write Capacity and Throttling
DynamoDB enforces certain limits to maintain optimal performance:
- Maximum Capacity per Partition: 3,000 read units per second and 1,000 write units per second.
- Read and Write Units: One read unit equals one strongly consistent read per second (or two eventually consistent reads) for an item up to 4 KB. One write unit equals one write per second for an item up to 1 KB.
These limits are crucial to understand when designing your retry logic to avoid throttling and ensure efficient data writes.
Implementing an Exponential Backoff Algorithm
When DynamoDB throws an error, an exponential backoff algorithm helps manage retries efficiently. This algorithm increases the wait time between successive retries, preventing your application from overwhelming the database.
Common Errors and Retry Logic
Here are some common errors you might encounter and whether they should be retried:
- ServiceUnavailable (HTTP 503)
- Message: DynamoDB is currently unavailable (temporary state).
- Retry: Yes
- InternalServerError (HTTP 500)
- Message: DynamoDB could not process your request.
- Retry: Yes
- ThrottlingException
- Message: Rate of requests exceeds the allowed throughput.
- Retry: Yes
- UnrecognizedClientException
- Message: The Access Key ID or security token is invalid.
- Retry: Yes
- ProvisionedThroughputExceededException
- Message: You exceeded your maximum allowed provisioned throughput.
- Retry: Yes
- RequestLimitExceeded
- Message: Throughput exceeds the current throughput limit for your account.
- Retry: Yes
- ItemCollectionSizeLimitExceededException
- Message: Collection size exceeded (for tables with a local secondary index).
- Retry: Yes
- LimitExceededException
- Message: Too many operations for a given subscriber.
- Retry: Yes
Sample Implementation in Node.js
Here is a sample implementation of an exponential backoff algorithm in Node.js using the AWS SDK:
const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB.DocumentClient();
async function batchWriteWithRetries(items) {
const maxRetries = 5;
const baseDelay = 50; // milliseconds
let attempt = 0;
let unprocessedItems = items;
while (attempt < maxRetries && unprocessedItems.length > 0) {
const params = {
RequestItems: {
'YourTableName': unprocessedItems.map(item => ({
PutRequest: {
Item: item
}
}))
}
};
try {
const response = await dynamoDB.batchWrite(params).promise();
unprocessedItems = response.UnprocessedItems['YourTableName'] || [];
if (unprocessedItems.length > 0) {
attempt++;
const delay = baseDelay * Math.pow(2, attempt);
await new Promise(resolve => setTimeout(resolve, delay));
}
} catch (error) {
console.error(`Batch write failed: ${error.message}`);
if (['ServiceUnavailable', 'InternalServerError',
'ThrottlingException', 'ProvisionedThroughputExceededException',
'RequestLimitExceeded',
'LimitExceededException'].includes(error.code)) {
attempt++;
const delay = baseDelay * Math.pow(2, attempt);
await new Promise(resolve => setTimeout(resolve, delay));
} else {
throw error;
}
}
}
if (unprocessedItems.length > 0) {
throw new Error('Max retries reached. Some items could not be processed.');
}
}
Detailed Explanation of the Code
- Setting Up AWS SDK:
- We import the AWS SDK and initialize the
DocumentClient
for DynamoDB operations.
- We import the AWS SDK and initialize the
- Defining
batchWriteWithRetries
Function:- This function accepts an array of items to be written to DynamoDB.
maxRetries
andbaseDelay
are defined to control the retry mechanism.
- Handling Unprocessed Items:
- The loop continues until all items are processed or the maximum number of retries is reached.
unprocessedItems
are the items that failed to write in the previous attempt.
- Batch Write Operation:
- The
batchWrite
method ofdynamoDB
is called with the provided items. - If there are unprocessed items in the response, they are retried with an increasing delay.
- The
- Error Handling:
- The code catches common DynamoDB errors and retries the operation with an exponential backoff.
- If an error is not related to temporary issues, it is thrown immediately.
- Final Check:
- If there are still unprocessed items after the maximum retries, an error is thrown.
Conclusion
Handling large data writes in DynamoDB requires careful planning and implementation of retry logic. By understanding DynamoDB’s capacity limits and using an exponential backoff algorithm, you can manage retries effectively and ensure your data is written successfully. This approach helps maintain your application's performance and reliability even under high load conditions.
Further Considerations
- Monitoring and Logging: Implement logging to monitor the performance of your batch write operations and identify any patterns in the errors.
- Scaling DynamoDB: If you frequently encounter throttling, consider increasing your table's provisioned throughput or switching to on-demand mode.
- Parallel Processing: For extremely large datasets, consider processing your data in parallel batches to improve throughput while managing retries.
By following these best practices, you can ensure efficient and reliable data handling in DynamoDB, even when dealing with large volumes of data.