Exponential Backoff Algorithm in Cypress
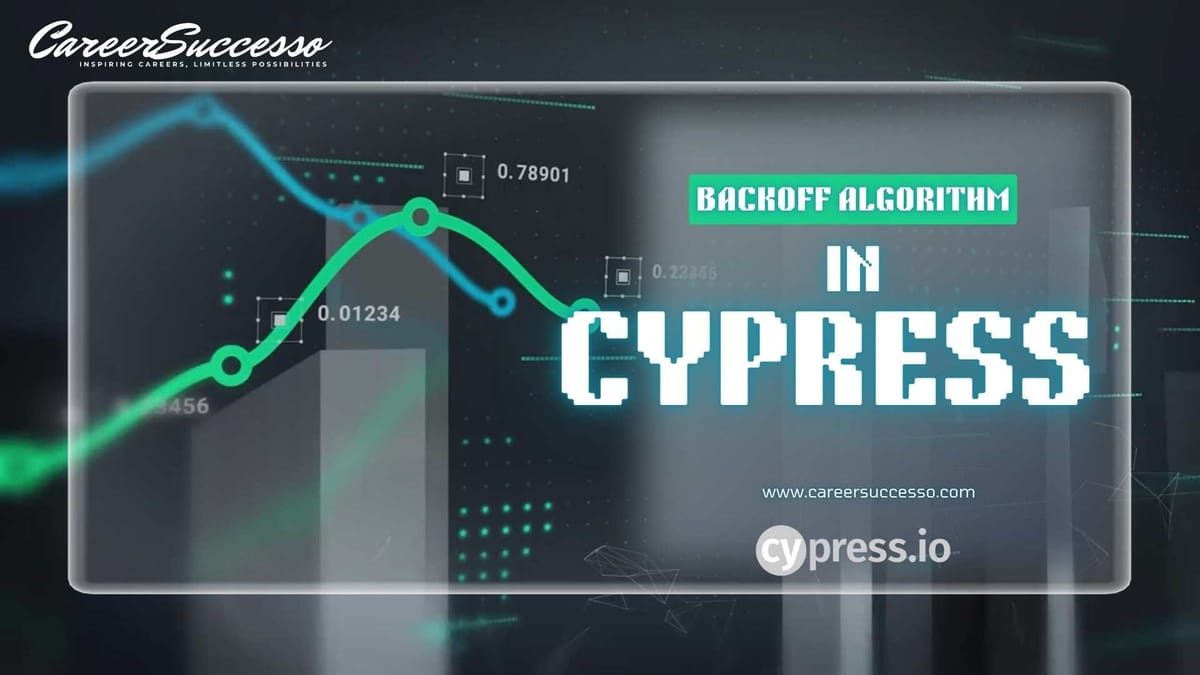
When performing automated tests or making repeated network requests, it's crucial to implement a strategy that prevents overwhelming the server and ensures efficient retries in case of transient failures. One effective approach is the exponential backoff algorithm. This guide will explore how to implement the exponential backoff algorithm in Cypress, a popular JavaScript-based end-to-end testing framework.
What is Exponential Backoff?
Exponential backoff is a retry strategy that increases the wait time between consecutive retry attempts exponentially. This approach helps to reduce the load on the server and increases the chances of successful retries by giving the server more time to recover from transient issues.
Why Use Exponential Backoff?
- Prevent Overload: Repeatedly hitting a server with rapid consecutive requests can cause further failures or slowdowns. Exponential backoff mitigates this by spacing out the retries.
- Improve Reliability: By allowing more time between retries, transient errors (like network issues or temporary server unavailability) have a higher chance of being resolved.
- Efficient Resource Use: It avoids unnecessary resource consumption by not attempting retries too quickly.
Implementing Exponential Backoff in Cypress
Let’s walk through how to implement the exponential backoff algorithm in Cypress. Suppose we want to check the status of a processing request.
Step-by-Step Implementation
- Initial Setup: Define the maximum number of retry attempts and create a function that will handle the retry logic.
const maxRetries = 20;
function checkProcessingStatus(attempt = 0) {
if (attempt > maxRetries) {
throw new Error('Processing timeout');
}
}
- Making the Request: Use Cypress's cy.request to make an HTTP GET request to check the processing status.
cy.request({
method: 'GET',
url: `${Cypress.env('baseUrl')`,
headers: {
Accept: 'application/json',
Authorization: `${authToken}`,
},
}).then((response) => {
expect(response.status).to.eq(200);
expect(response.body).to.be.an('object');
const status = response.body.status;
}
- Checking Status: Evaluate the processing status in the response. If the status is not as expected, proceed to retry.
if (status === 'error') {
expect(status).to.eq('error');
cy.log('Processing failed as expected');
} else {
// Exponential Backoff with Jitter
const nextInterval = Math.min(10000 * Math.pow(2, attempt) + Math.random() * 1000);
cy.wait(nextInterval).then(() => {
checkProcessingStatus(attempt + 1);
});
}
- Recursive Retry: The function checkProcessingStatus recursively calls itself, incrementing the attempt count until the maximum number of retries is reached or the processing status is as expected.
checkProcessingStatus();
Complete Example in Cypress
Here is the complete code snippet integrating the exponential backoff algorithm in Cypress:
describe('Processing Status Check', () => {
it('Checks the processing status with exponential backoff', () => {
const authToken = Cypress.env('authToken');
function checkProcessingStatus(attempt = 0) {
if (attempt > maxRetries) {
throw new Error('Processing timeout');
}
cy.request({
method: 'GET',
url: `${Cypress.env('baseUrl')}`,
headers: {
Accept: 'application/json',
Authorization: `Bearer ${authToken}`,
},
}).then((response) => {
expect(response.status).to.eq(200);
expect(response.body).to.be.an('object');
const status = response.body.status;
if (status === 'error') {
expect(status).to.eq('error');
cy.log('Processing failed as expected');
} else {
const nextInterval = Math.min(10000 * Math.pow(2, attempt) + Math.random() * 1000);
cy.wait(nextInterval).then(() => {
checkProcessingStatus(attempt + 1);
});
}
});
}
checkProcessingStatus();
});
});
});
Conclusion
Implementing the exponential backoff algorithm in Cypress enhances the reliability and efficiency of your automated tests. By spacing out retries and adding random delays, you can reduce the load on your server and increase the likelihood of successful operations in the presence of transient issues. This approach is especially useful for network requests and other operations that may fail temporarily.