Reusing Dynamic Values in Cypress Tests: Store Once, Reuse Anywhere
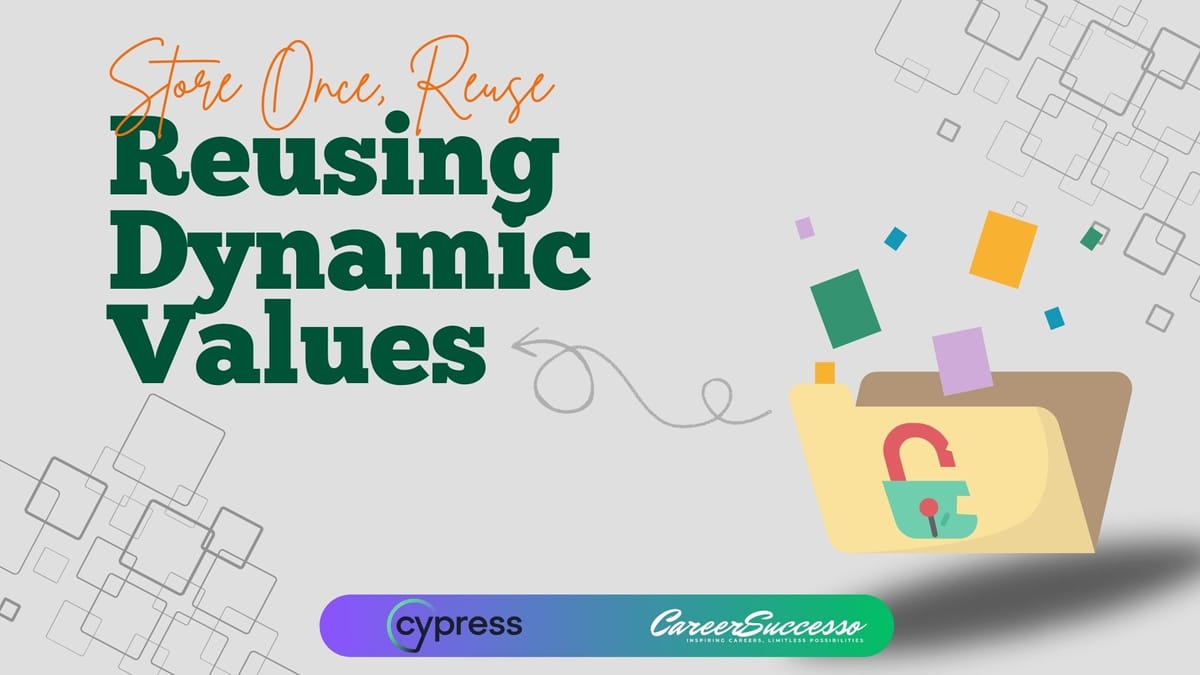
In test automation, maintaining and reusing state between test cases is essential for creating efficient, end-to-end workflows. When writing Cypress tests that interact with APIs, it's common to receive dynamic data from a response—such as an ID, token, or reference number—that you'll need to use in a later request. Instead of hardcoding or repeating logic, Cypress allows you to store these values temporarily during the test run and reuse them wherever needed.
Let’s explore how to do this in a clean and efficient way.
Why Store Values Dynamically?
Many real-world scenarios involve multi-step processes where the output of one step is the input for the next. For instance:
- A request may return an identifier (id) that’s required for the next API call.
- A system may provide an access token that must be included in subsequent headers.
- You might need to retrieve the status of a resource using a previously returned reference.
By reusing these variables, you:
Reduce redundancy: Avoid recreating the same data in every test.
Improve performance: Skip repetitive setup steps.
Mimic real-world workflows: Simulate multi-step user journeys.
01.Use Cypress.env() to Store and Reuse Data
Cypress provides a convenient way to store values in memory using Cypress.env() during a test run.
Store the Value
When you receive a value from an API response, you can store it like this:
cy.request('POST', 'your-api-url', requestBody).then((response) => {
const dynamicId = response.body.id;
Cypress.env('dynamicId', dynamicId); // Save it temporarily
});
Reuse It in Another Request
You can then access that value in a later test step:
cy.request({
method: 'GET',
url: `your-api-url/${Cypress.env('dynamicId')}`,
headers: {
Authorization: `Bearer yourAccessToken`,
},
}).then((response) => {
expect(response.status).to.eq(200);
// more assertions
});
Example with Tokens
Here’s how to store an access token received from an authorization request:
cy.request('POST', 'token-url', credentials).then((response) => {
const token = response.body.access_token;
Cypress.env('accessToken', token); // Stored in memory
});
Use it later:
cy.request({
method: 'GET',
url: 'api/secure-data',
headers: {
Authorization: `Bearer ${Cypress.env('accessToken')}`, // Used here
},
});
02.Using Test-Specific Variables
For data needed only within a single test file, use closures (e.g., let variables).
Example: Reusing a Resource ID
describe('Reusing ResourceID Tests', () => {
let resourceId; // Accessible across all tests in this describe block
it('Creates a Resource', () => {
cy.request('/resources', { method: 'POST' }).then((response) => {
resourceId = response.body.id; // Assign ID
});
});
it('Validates the Resource', () => {
cy.request(`/resources/${resourceId}`).then((response) => {
expect(response.status).to.eq(200);
});
});
});
03. External Storage (Files, Databases)
For cross-suite or cross-run persistence, save data to files (e.g., JSON) or databases. Use this sparingly, as it introduces external dependencies.
Good Practices
- Use Cypress.env() for temporary values within the same test suite.
- Do not use Cypress.env() as a way to persist values between test files.
- Store static configuration in cypress.env.json, but store dynamic values using Cypress.env() at runtime.
- Clean Up State: Delete test data after runs (e.g., in after hooks).
- Avoid Hardcoding Values: Use environment variables or configuration files for URLs, keys, etc.
- Handle Asynchronous Flows: Use cy.wait() or retry logic if systems need time to process data.
Summary
Storing dynamic data during test execution helps avoid hardcoding and keeps your Cypress tests clean and reliable. By leveraging Cypress environment variables, closures, or external storage, you can build robust test suites that minimize redundancy and maximize efficiency. Always prioritize clarity and maintainability—use descriptive variable names and document shared state dependencies. This approach improves test reusability, enhances readability, and supports more complex workflows in your automation suite.
👉 For more articles like this, visit my site: https://careersuccesso.com/