Sequential Multi-User Testing in a Single Test with Cypress
Multi-User Testing is a software testing approach that evaluates the performance, scalability, and robustness of an application by…
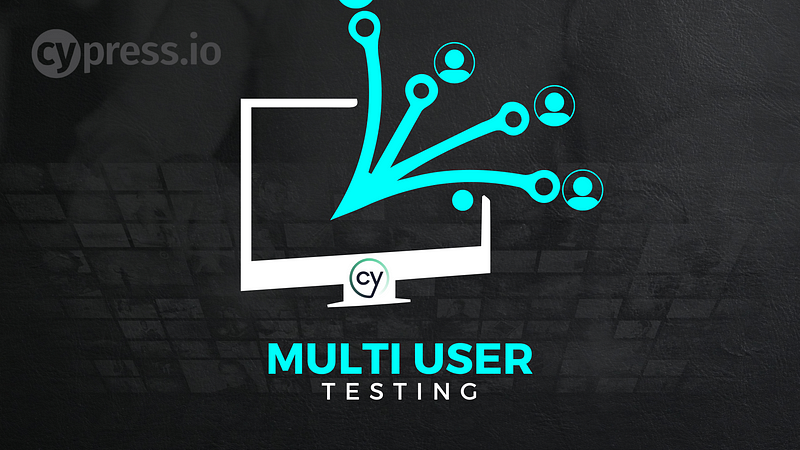
Multi-User Testing is a software testing approach that evaluates the performance, scalability, and robustness of an application by simulating interactions from multiple users. Multi-user” signifies the concurrent or sequential engagement of several virtual users with the application to assess its responsiveness, resource utilization, and overall functionality under realistic conditions.
Modern web applications often require robust testing procedures to ensure a seamless user experience. Testing how well an application handles concurrent user interactions is crucial for ensuring a robust user experience. While Cypress doesn’t inherently support controlling more than one open browser at a time, there are clever workarounds.
In this article, I’ll embark on a journey to explore two distinctive approaches — one utilizing Cypress sessions and the other leveraging custom commands. By the end, you’ll possess a comprehensive toolkit for sequential multi-user testing that can uncover potential issues and ensure a flawless user experience.
Method 1:Cypress Sessions for Multi-User Testing:
Cypress offers a unique feature called sessions, allowing us to manage different user contexts within a single test script. The following code exemplifies how Cypress sessions can be employed to verify user and admin logins:
describe('Multi-User Testing', () => {
it('Verifying Admin login and Users Login', () => {
// User 1 login
cy.session('user', () => {
cy.visit('url', { failOnStatusCode: false });
cy.get('#email').type('user_email');
cy.get('#password').type('user_password');
cy.get('#submit').click();
}, { cacheAcrossSpecs: true });
// Admin login
cy.session('Admin', () => {
cy.visit('url/admin', { failOnStatusCode: false });
cy.get('#adminemail').type('admin_email');
cy.get('#adminpassword').type('admin_password');
cy.get('#adminsubmit').click();
}, { cacheAcrossSpecs: true });
// User 2 login
cy.session('user2', () => {
cy.visit('url', { failOnStatusCode: false });
cy.get('#email').type('user2_email');
cy.get('#password').type('user2_password');
cy.get('#submit').click();
});
});
});
Breaking Down the Code:
User 1 Login: Utilizing cy.session(‘user’), we simulate the login scenario for a regular user.
Admin Login: Similarly, cy.session(‘Admin’) orchestrates the login process for an administrator.
User 2 Login: Lastly, we demonstrate how to test another user scenario using cy.session(‘user2’).
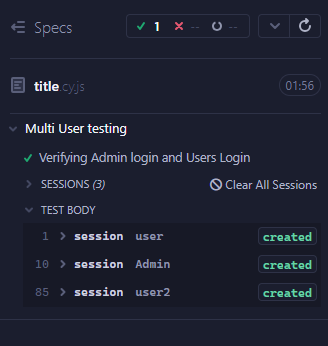
Method 2:Custom Commands in commands.js:
To get started, enhance your Cypress commands by adding custom commands for each user login. In your `commands.js` file, implement functions like `LoginUser` to facilitate easy login for each user:
Cypress.Commands.add('LoginUser', (username, password) => {
cy.visit('url', { failOnStatusCode: false });
cy.get('#email').type(username);
cy.get('#-password').type(password);
cy.get('#submit').click();
});
Creating the Test Script:
Now, in your test script (e.g., `cy.js`), structure your tests using Cypress’s `describe` and `it` blocks. Define user data for each scenario, such as user1Data and user2Data:
describe('Multi User Login Testing', () => {
const user1Data = {
username: 'user1',
password: 'password1'
};
const user2Data = {
username: 'user2',
password: 'password2'
};
it('Verify login user 1 and user 2', () => {
// Test for user 1
cy.LoginUser(user1Data.username, user1Data.password);
cy.clearCookies(); // Clear cookies
cy.clearLocalStorage(); // Clear local storage
// Test for user 2
cy.LoginUser(user2Data.username, user2Data.password);
cy.clearCookies(); // Clear cookies
cy.clearLocalStorage(); // Clear local storage
});
});
Both custom commands and Cypress sessions serve as suitable methods for sequential multi-user testing in Cypress. Custom commands enhance test readability and maintainability by encapsulating repetitive actions, while Cypress sessions provide a structured approach to manage different user contexts within a single test script. By leveraging these methods, testers can simulate diverse user interactions efficiently, ensuring that their web applications deliver a seamless experience for all users. Incorporate these approaches into your testing workflow to elevate the quality and reliability of your web applications.