Simplifying Retry Logic with AWS Step Functions and Lambda
In serverless applications, handling retries is a common challenge. AWS Step Functions, when combined with AWS Lambda and the AWS Cloud…
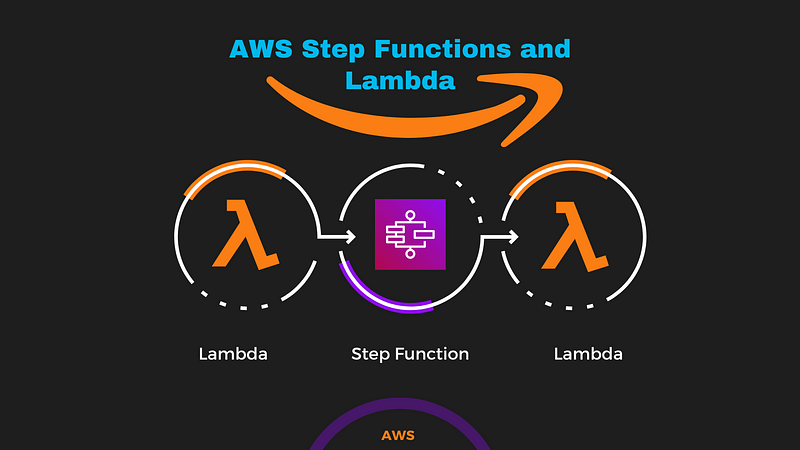
In serverless applications, handling retries is a common challenge. AWS Step Functions, when combined with AWS Lambda and the AWS Cloud Development Kit (CDK), provides an elegant solution for managing and automating retry workflows. In this blog post, we’ll explore a simple example of creating a retry mechanism using AWS Step Functions and Lambda.
AWS CDK for Step Function and Lambda:
To get started, let’s take a look at a basic AWS CDK code snippet that defines a Step Function with three states: invoking a Lambda function (submitJob
), waiting for 5minutes (wait5Minutes
), and retrying another Lambda function (retryDelete
).
const submitJob = new tasks.LambdaInvoke(this, 'Submit Job', {
lambdaFunction: lambdafn1,
});
const wait5Minutes = new sfn.Wait(this, 'Wait 5Minutes', {
time: sfn.WaitTime.duration(Duration.minutes(5)),
});
const retryDeleteInput = new tasks.LambdaInvoke(this, 'Retry Delete', {
lambdaFunction: lambdafn2,
});
const definition = submitJob
.next(wait5Minutes)
.next(retryDelete);
const stateMachine = new sfn.StateMachine(this, 'RetryStateMachine', {
definition: definition,
stateMachineName: "string_value",
});
This CDK code defines a state machine named RetryStateMachine
with a sequence of steps. First, it invokes a Lambda function (submitJob
), then waits for 5 minutes, and finally retries another Lambda function (retryDelete
).
Handling Errors with AWS Step Functions
Errors can occur during the execution of Lambda functions. AWS Step Functions allows you to gracefully handle errors by defining error-catching mechanisms within the state machine. If the first Lambda function (submitJob
) encounters an error, it will be captured, and the execution will proceed to the next state, wait5Minutes
.In AWS Step Functions, the output of a state becomes the input for the next state.
Initiating the Retry Workflow:
Now, let’s address the scenario where an error occurs, and we want to initiate a retry workflow asynchronously. The following code demonstrates how to start the execution of the defined state machine in the event of an error.
import { SFNClient, StartExecutionCommand } from "@aws-sdk/client-sfn"; // ES Modules import
const client = new SFNClient(config);
const input = { // StartExecutionInput
stateMachineArn: "STRING_VALUE", // required
name: "STRING_VALUE",
input: "STRING_VALUE",
traceHeader: "STRING_VALUE",
};
const command = new StartExecutionCommand(input);
const response = await client.send(command);
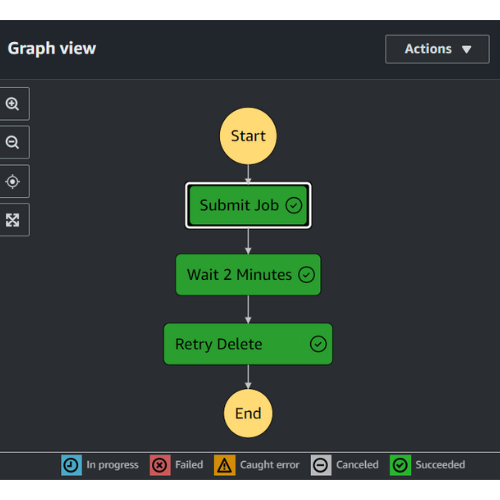
Conclusion
AWS Step Functions, in combination with AWS Lambda and CDK, provides a powerful way to handle retries in serverless workflows. By defining state machines and leveraging asynchronous execution, you can create robust and scalable retry mechanisms for your serverless applications.
Explore more about AWS Step Functions and Lambda for your serverless workflows and simplify your error handling with ease.