Streamlining Localization (internationalization) in React Apps with i18n and Context API
Localization is a pivotal aspect of crafting inclusive and user-friendly React applications, especially when targeting diverse global audiences. In this guide, we’ll explore how to seamlessly implement localization using the i18n library alongside
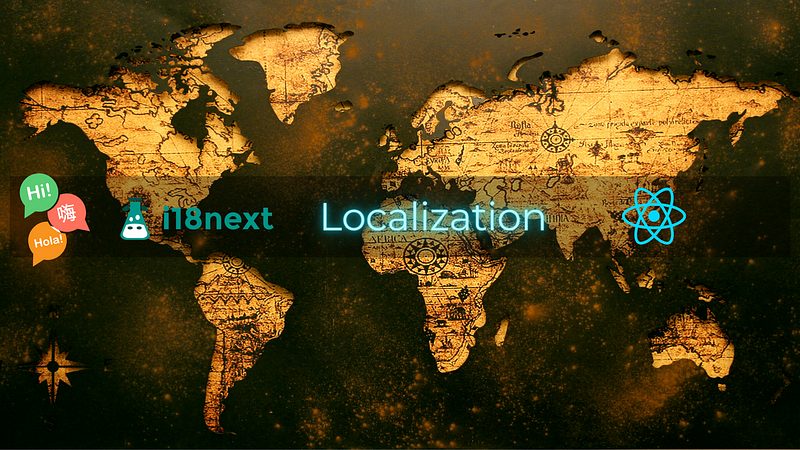
Introduction:
Localization is a pivotal aspect of crafting inclusive and user-friendly React applications, especially when targeting diverse global audiences. In this guide, we’ll explore how to seamlessly implement localization using the i18n library alongside the robust Context API, leveraging TypeScript for enhanced type safety and code integrity.
Getting Started with i18n in React:
To kickstart our journey, we first need to set up the i18n library within our React application. Begin by installing the essential packages:
npm install i18next react-i18next
Next, let’s configure i18n in our app by creating a dedicated file named i18n.ts :
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
i18n
.use(initReactI18next)
.init({
resources: {
en: {
translation: {
hello: 'Hello',
// Additional translations can be added here
},
},
// Add translations for other languages as needed
},
lng: 'en',
fallbackLng: 'en',
interpolation: {
escapeValue: false,
},
});
export default i18n;
This setup initializes i18n with English as the default language and allows for seamless expansion to accommodate multiple languages.
Establishing a Language Context:
Central to our localization strategy is the creation of a Language Context using React’s Context API. This context enables the management of the current language state across the entire application. Let’s define this context in a file named LanguageContext.tsx
:
import React, { createContext, useContext, useState } from 'react';
interface LanguageContextType {
language: string;
setLanguage: React.Dispatch<React.SetStateAction<string>>;
}
const LanguageContext = createContext<LanguageContextType | undefined>(undefined);
export const LanguageProvider: React.FC = ({ children }) => {
const [language, setLanguage] = useState('en');
return (
<LanguageContext.Provider value={{ language, setLanguage }}>
{children}
</LanguageContext.Provider>
);
};
export const useLanguage = () => {
const context = useContext(LanguageContext);
if (!context) {
throw new Error('useLanguage must be used within a LanguageProvider');
}
return context;
};
This context empowers developers to effortlessly manage language changes and updates throughout the application.
Integrating Localization in Components:
With our infrastructure in place, let’s integrate i18n and the Language Context into our components. Consider a foundational component such as the Top Bar:
import React from 'react';
import { useTranslation } from 'react-i18next';
import { useLanguage } from './LanguageContext';
const TopBar: React.FC = () => {
const { t } = useTranslation();
const { language, setLanguage } = useLanguage();
const changeLanguage = (lng: string) => {
setLanguage(lng);
};
return (
<div>
<button onClick={() => changeLanguage('en')}>English</button>
<button onClick={() => changeLanguage('fr')}>French</button>
{/* Additional language buttons can be added here */}
<div>{t('hello')}</div>
</div>
);
};
export default TopBar;
This component seamlessly integrates language selection functionality while providing access to translations via the useTranslation
hook.
Incorporating TopBar into Pages:
The Top Bar component can be effortlessly integrated into various pages of the application:
import React from 'react';
import TopBar from './TopBar';
const HomePage: React.FC = () => {
return (
<div>
<TopBar />
<h1>Home Page</h1>
{/* Additional home page content can be added here */}
</div>
);
};
export default HomePage;
Conclusion:
In this comprehensive guide, we’ve demonstrated the seamless implementation of localization in React applications using the i18n library and the Context API. By leveraging these powerful tools in conjunction with TypeScript, developers can create dynamic and inclusive applications that cater to diverse linguistic preferences. Embrace the power of localization to enhance user experience and foster global accessibility within your React projects.